일 | 월 | 화 | 수 | 목 | 금 | 토 |
---|---|---|---|---|---|---|
1 | 2 | 3 | 4 | 5 | ||
6 | 7 | 8 | 9 | 10 | 11 | 12 |
13 | 14 | 15 | 16 | 17 | 18 | 19 |
20 | 21 | 22 | 23 | 24 | 25 | 26 |
27 | 28 | 29 | 30 |
- 파이토치
- 미디언 필터링
- MFC 프로그래밍
- c++공부
- 모두의 딥러닝 예제
- pytorch
- 김성훈 교수님 PyTorch
- 케라스 정리
- C언어 공부
- c++
- 파이토치 강의 정리
- 컴퓨터 비전
- tensorflow 예제
- pytorch zero to all
- Pytorch Lecture
- 파이토치 김성훈 교수님 강의 정리
- c언어 정리
- 팀프로젝트
- TensorFlow
- 딥러닝 스터디
- 해리스 코너 검출
- 모두의 딥러닝
- 딥러닝 공부
- c언어
- 영상처리
- matlab 영상처리
- 골빈해커
- object detection
- 가우시안 필터링
- 딥러닝
- Today
- Total
ComputerVision Jack
[모두의 딥러닝 Chapter07] 본문
[07-1 learning_rate and evalutation]
learning rate 중요성
learning_rate을 바꿔 가면서 다양하게 실험해본다.
x_data = [[1, 2, 1],
[1, 3, 2],
[1, 3, 4],
[1, 5, 5],
[1, 7, 5],
[1, 2, 5],
[1, 6, 6],
[1, 7, 7]]
y_data = [[0, 0, 1],
[0, 0, 1],
[0, 0, 1],
[0, 1, 0],
[0, 1, 0],
[0, 1, 0],
[1, 0, 0],
[1, 0, 0]]
#x와 y 데이터 준비, 데이터 shape을 보면 다분류기라는 것을 알 수 있다.
x_test = [[2, 1, 1],
[3, 1, 2],
[3, 3, 4]]
y_test = [[0, 0, 1],
[0, 0, 1],
[0, 0, 1]]
#학습된 모델의 일반화 검사를 위한 test_set 데이터 만들기
X = tf.placeholder("float", [None, 3])
Y = tf.placeholder("float", [None, 3])
W = tf.Variable(tf.random_normal([3, 3]))
b = tf.Variable(tf.random_normal([3]))
# X데이터와 y데이터 가중치 편햔을 준비한다
hypothesis = tf.nn.softmax(tf.matmul(X, W) + b)
cost = tf.reduce_mean(-tf.reduce_sum(Y * tf.log(hypothesis), axis = 1))
optimizer = tf.train.GradientDescentOptimizer(learning_rate = 0.1).minimize(cost)
# 3단 공식을 설정한다. 모델을 정의하고 손실 함수를 생성하고 최적화를 실행한다.
다양하게 learning_rate을 설정하여 실행해 본다. 0.01, 1e-5 ..
prediction = tf.argmax(hypothesis , 1)
is_correct = tf.equal(prediction, tf.argmax(Y, 1))
accuracy = tf.reduce_mean(tf.cast(is_correct, tf.float32))
#모델의 정확도를 예측할 수 있게 준비한다.
with tf.Session() as sess:
sess.run(tf.global_variables_initializer())
for step in range(201):
cost_val, W_val, _ = sess.run([cost, W, optimizer], feed_dict = {X: x_data, Y: y_data})
print(step, cost_val, W_val)
print("Prediction : ", sess.run(prediction, feed_dict = {X: x_test}))
print("Accuracy : ", sess.run(accuracy, feed_dict = {X: x_test, Y: y_test}))
#feed_dict으로 데이터를 던져주면서 결과를 확인한다.
[07-2 linear_regression_without_min_max]
자료를 정규화 하지 않을 경우
xy = np.array([[828.659973, 833.450012, 908100, 828.349976, 831.659973],
[823.02002, 828.070007, 1828100, 821.655029, 828.070007],
[819.929993, 824.400024, 1438100, 818.97998, 824.159973],
[816, 820.958984, 1008100, 815.48999, 819.23999],
[819.359985, 823, 1188100, 818.469971, 818.97998],
[819, 823, 1198100, 816, 820.450012],
[811.700012, 815.25, 1098100, 809.780029, 813.669983],
[809.51001, 816.659973, 1398100, 804.539978, 809.559998]])
#위 데이터의 모습에서 데이터 간의 value가 많은 차이를 갖고 있다고 생각할 수 있다
모델에 데이터를 넣어줄 땐, 값의 차이가 크지 않고 고른 값을 가질 수 있도록 정규화 하여 넣어야 한다.
우선, 정규화를 하지 않고 넣는다.
hypothesis = tf.matmul(X, W) + b
#linear model을 사용하여 돌리면 얼마 지나지 않아 모델이 값을 예측할수 없다고
nan값을 내보내게 된다.
[07-3 linear_regression_min_max]
정규화 함수 추가
이제 정규화 함수를 추가하여 xy데이터를 한번 정제한다.
def min_max_scalar(data):
numerator = data - np.min(data, 0)
denominator = np.max(data, 0) - np.min(data, 0)
return numerator / (denominator + 1e-7)
xy = min_max_scalar(xy)
print(xy)
#데이터가 0~1 사이의 값으로 정규화가 진행되고, 값의 차이가 크지 않다.
x_data = xy[:, 0: -1]
y_data = xy[:, [-1]]
#x와 y의 데이터를 자른 후, 다시 모델에 학습하면 계속 학습이 진행되는 것을 알 수 있다.
nan값이 더이상 발생하지 않는다.
[07-4 mnist_introduction]
mnist자료형
손끌씨 데이터 이미지인 mnist를 소개한다. mnist는 0 ~ 9까지 손글씨가 쓰여있는 이미지 파일이다.
또한 각 이미지 파일의 크기는 28 * 28 픽셀이다.
데이터의 입력으로 우린 영상의 전체 (28 * 28)을 사용한다.
from tensorflow.examples.tutorials.mnist import input_data
mnist = input_data.read_data_sets("MNIST_data/", one_hot=True)
#mnist자료형을 읽어온다.
nb_classes = 10
#최종 분류 클래스는 0~9 총 10개이다.
X = tf.placeholder(tf.float32, [None, 784])
Y = tf.placeholder(tf.float32, [None, nb_classes])
#영상을 통으로 입력으로 사용하기 때문에 [none, 28 * 28] shape이 된다.
Y 또한 출력 클래스의 맞게 조절한다.
W = tf.Variable(tf.random_normal([784, nb_classes]))
b = tf.Variable(tf.random_normal([nb_classes]))
#가중치와 편향 설정.
hypothesis = tf.nn.softmax(tf.matmul(X, W) + b)
cost = tf.reduce_mean(-tf.reduce_sum(Y * tf.log(hypothesis), axis = 1))
train = tf.train.GradientDescentOptimizer(learning_rate = 0.1).minimize(cost)
#모델과 손실함수, 경사하강법을 정의한다.
is_correct = tf.equal(tf.argmax(hypothesis , 1), tf.argmax(Y, 1))
accuracy = tf.reduce_mean(tf.cast(is_correct, tf.float32))
#정확도 검출작업 준비
num_epochs = 15
batch_size = 100
num_iterations = int(mnist.train.num_examples / batch_size)
#data set이 클경우 한번에 모델에 넣어서 학습하기 보단, batch_size로 dataset을 자른후 입력으로 넣는다.
만약 데이터가 1500개가 있었다고 한다면 batch_size = 100으로 했을 때, 1500 / 100 총 15번 반복을 돌아야 한다.
그리고, 15번의 반복이 돌아야 1회 학습이 되었다 하여 1epochs라고 한다.
with tf.Session() as sess:
sess.run(tf.global_variables_initializer())
for epoch in range(num_epochs):
avg_cost = 0
for i in range(num_iterations):
batch_xs, batch_ys = mnist.train.next_batch(batch_size)
_, cost_val = sess.run([train, cost], feed_dict = {X: batch_xs, Y: batch_ys})
avg_cost += cost_val / num_iterations
print("Epoch : {:04d}, Cost : {:.9f}".format(epoch + 1, avg_cost))
print("Learing finised")
#Test the model using test sets
print("Accuracy : ", accuracy.eval(session = sess, feed_dict = {X: mnist.test.images, Y: mnist.test.labels}),)
# Get one and predict
r = random.randint(0, mnist.test.num_examples - 1)
print("Label : ", sess.run(tf.argmax(mnist.test.labels[r : r + 1], 1)))
print("Prediction : ", sess.run(tf.argmax(hypothesis, 1), feed_dict = {X: mnist.test.images[r : r + 1]}),)
plt.imshow(
mnist.test.images[r : r + 1].reshape(28, 28),
cmap = "Greys",
interpolation="nearest",
)
plt.show()
#학습을 시키고 matplotlib 라이브러리를 사용하여 결과를 출력하면 화면에 표시됨을 알 수 있다.
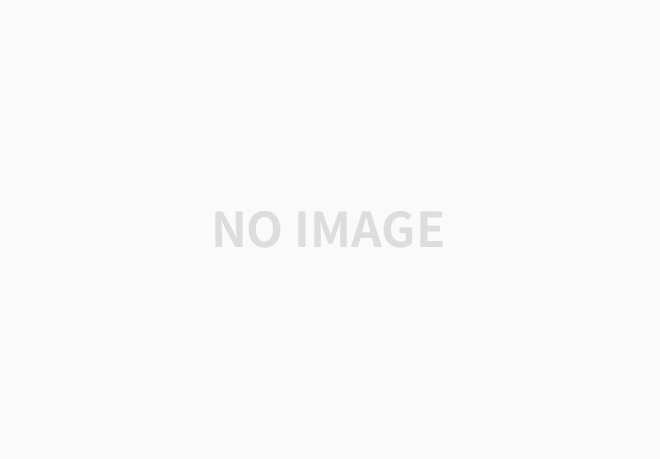
'DeepLearning > DL_ZeroToAll' 카테고리의 다른 글
[모두의 딥러닝 Chapter09] (0) | 2020.01.21 |
---|---|
[모두의 딥러닝 Chapter08] (0) | 2020.01.20 |
[모두의 딥러닝 Chapter06] (0) | 2020.01.17 |
[모두의 딥러닝 Chapter05] (0) | 2020.01.16 |
[모두의 딥러닝 Chapter04] (2) | 2020.01.15 |